In this section we will walk through the writing of some C# sample code.
Establishing a connection to the MiX.Integrate APIReturn data from the API
Basic error handling
Full sample code
Useful links
Establishing a connection to the MiX.Integrate API
To begin the login process you will need to create a new asynchronous class with two variables that will hold the Base API URL and the Client settings (username, password, Organisation Id, scope, ClientId and ClientSecret)
Create and assign 3 variables to the class which will be loaded from the App.Config.
APIBaseURL
The MiX.Integrate Base URL which will load the ApiUrl from the App.Config
idServerResourceOwnerClientSettings
The variable that will load the client settings from the App.Config
organisationGroupId
The Organisation Id which will be loaded from the App.Config
using MiX.Integrate.Api.Client; using MiX.Integrate.Shared.Entities.Groups; using System; using System.Configuration; usingSystem.Threading.Tasks; namespace MiXIntegrateLoginSample { class Program { static void Main(string[] args) { Login().Wait(); } private static async Task Login() { var apiBaseUrl = ConfigurationManager.AppSettings["ApiURL"]; var idServerResourceOwnerClientSettings = new IdServerResourceOwnerClientSettings() { }; } } }
At this point you will notice that idServerResourceOwnerClientSettings
is unreferenced, to add this reference,
at the very top of your application add "using MiX.Intigrate.Api.Client;"
this will add the using statement code, and remove the underlining.
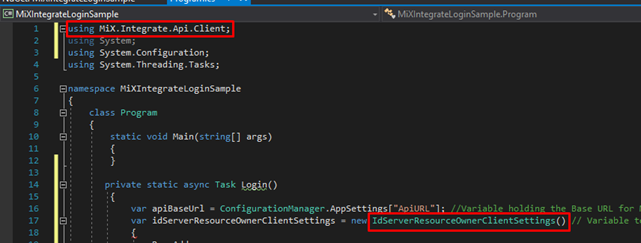
You can now continue to initialize the objects to set the values of properties of the idServerResourceOwnerClientSettings object variable;
BaseAddress
The Identity Servers base URL
ClientId
The Client Id assigned to the application
ClientSecret
The Client Secret associated with the Client Id
UserName
The user's MiX Fleet Manager username
Password
The user's MiX Fleet Manager password
Scopes
The scope of the Connection
Once the Object has been defined. The last property (organisationGroupId
) is set.
The final code snippet should look like the below:
** organisationGroupId is no longer the old 3 to 4 digit FM orgID, this is now the 64bit GroupId, this can be found by making a call to the GET /api/organisationgroups call to return a list of organisations available to the login.
private static async Task Login() { var apiBaseUrl = ConfigurationManager.AppSettings["ApiURL"]; var idServerResourceOwnerClientSettings = new IdServerResourceOwnerClientSettings() { BaseAddress = ConfigurationManager.AppSettings["IdentityServerBaseAddress"], ClientId = ConfigurationManager.AppSettings["IdentityServerClientId"], ClientSecret = ConfigurationManager.AppSettings["IdentityServerClientSecret"], UserName = ConfigurationManager.AppSettings["IdentityServerUserName"], Password = ConfigurationManager.AppSettings["IdentityServerPassword"], Scopes = ConfigurationManager.AppSettings["IdentityServerScopes"] }; var organisationGroupId = long.Parse(ConfigurationManager.AppSettings["OrganisationGroupId"]); }
Return data from the API
In the below code snippet, we continue from the previous section, and add in code to display the login status, along with some details of the logged in account and basic error handling.
To begin we will need to create a new asynchronous class called GetGroupSummary
, and this class will get the following:
OrganisationGroupId
The Organisation Id
apiBaseURL
The Base URL of the MiX.Integrate API
idServerResourceOwnerClientSettings
All the Identity Server client details
We first write to the console to inform the user that the organisations details are being retrieved, and then write to two variables:
GroupsClient
holds the client details stored in idServerResourceOwnerClientSettings
Group
Stores the Organisation Id
And then we return the group variable so that we can extract this data for display.
private static async Task<GroupSummary> GetGroupSummary(long organisationGroupId, string apiBaseUrl, IdServerResourceOwnerClientSettings idServerResourceOwnerClientSettings) { Console.WriteLine("Retrieving Organisation details"); var groupsClient = new GroupsClient(apiBaseUrl, idServerResourceOwnerClientSettings); var group = await groupsClient.GetSubGroupsAsync(organisationGroupId); return group; }
Back within our Login
method we add the following section of code to display a few details of the user and Organisation.
For the below sample, I first clear the console of any previous messages using Console.Clear();
We can then write a few lines to the console window using Console.WriteLine("");
and I have chosen to return a login status message, organisation name, clientId and MiX Fleet Manager username. Along with a few filler lines for display purpose and ended with a Console.ReadKey();
which will allow the application to close on any key press.
{ var group = await GetGroupSummary(organisationGroupId, apiBaseUrl, idServerResourceOwnerClientSettings); Console.Clear(); Console.WriteLine("Login Successful"); Console.WriteLine("##############################################"); Console.WriteLine("Organisation Name : {0}", group.Name); Console.WriteLine("ClientId : {0}", idServerResourceOwnerClientSettings.ClientId); Console.WriteLine("Username : {0}", idServerResourceOwnerClientSettings.UserName); Console.WriteLine("##############################################"); Console.WriteLine("Press any key to continue..."); Console.ReadKey(); }
At this point our application can connect to the Identity Server, validate the client and log the MiX Fleet Manager user in. We can run the application, and if successful you should see a return like the below:
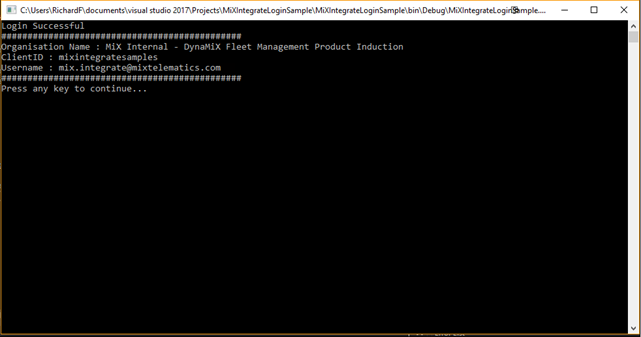
Basic error handling
Even though our application is now able to successfully connect to the API servers and validate our credentials. We still need some basic error handling should there be any failures. In this section, we will again continue to build on our sample application and add in some Try, Catch, basic error handling.
We begin by creating a new class: private static void PrintException(Exception ex)
at the end of our main application class. In this class, we write the exception to the console again using Console.WriteLine("ex.Message");
followed by a simple if statement to check if the Inner Exception is not null, and if so, to print the inner exception, the code looks like the below:
private static void PrintException(Exception ex) { Console.WriteLine(ex.Message); if (ex.InnerException != null) PrintException(ex.InnerException); }
Now that the application is configured to catch the errors and pass them to the console, we need to add try and catch statements in the sections of code we would like to be able to manage exceptions.
This would be within our Login method, where we attempt to return the login variables, we need to modify the existing code to contain a try, catch and finally.
{ try { var group = await GetGroupSummary(organisationGroupId, apiBaseUrl, idServerResourceOwnerClientSettings); Console.Clear(); Console.WriteLine("Login Successful"); Console.WriteLine("##############################################"); Console.WriteLine("Organisation Name : {0}", group.Name); Console.WriteLine("ClientId : {0}", idServerResourceOwnerClientSettings.ClientId); Console.WriteLine("Username : {0}", idServerResourceOwnerClientSettings.UserName); Console.WriteLine("##############################################"); } catch (Exception ex) { Console.WriteLine(""); Console.WriteLine("LOGIN UNSUCCESSFUL!"); Console.WriteLine("##############################################"); Console.WriteLine("Unexpected error:"); Console.WriteLine("##############################################"); PrintException(ex); } finally { Console.WriteLine("Press any key to continue..."); Console.ReadKey(); } }
If we now change the MiX Fleet Manager Users login in our App.Config we can force an error, and on running the application should see the below error:
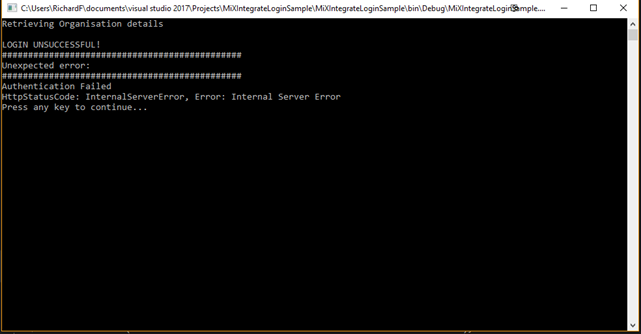
Remember to correct the password in the App.config file, and save the project.
Your application is now complete, and able to connect and authenticate a user.
Full sample code
Below is the complete code from the sample application we have just written.
using MiX.Integrate.Api.Client; using MiX.Integrate.Shared.Entities.Groups; using System; using System.Configuration; using System.Threading.Tasks; namespace MiXIntegrateLoginSample { class Program { static void Main(string[] args) { Login().Wait(); } private static async Task Login() { var apiBaseUrl = ConfigurationManager.AppSettings["ApiUrl"]; var idServerResourceOwnerClientSettings = new IdServerResourceOwnerClientSettings() { BaseAddress = ConfigurationManager.AppSettings["IdentityServerBaseAddress"], ClientId = ConfigurationManager.AppSettings["IdentityServerClientId"], ClientSecret = ConfigurationManager.AppSettings["IdentityServerClientSecret"], UserName = ConfigurationManager.AppSettings["IdentityServerUserName"], Password = ConfigurationManager.AppSettings["IdentityServerPassword"], Scopes = ConfigurationManager.AppSettings["IdentityServerScopes"] }; var organisationGroupId = long.Parse(ConfigurationManager.AppSettings["OrganisationGroupId"]); { try { var group = await GetGroupSummary(organisationGroupId, apiBaseUrl, idServerResourceOwnerClientSettings); Console.Clear(); Console.WriteLine("Login Successful"); Console.WriteLine("##############################################"); Console.WriteLine("Organisation Name : {0}", group.Name); Console.WriteLine("ClientId : {0}", idServerResourceOwnerClientSettings.ClientId); Console.WriteLine("Username : {0}", idServerResourceOwnerClientSettings.UserName); Console.WriteLine("##############################################"); } catch (Exception ex) { Console.WriteLine(""); Console.WriteLine("LOGIN UNSUCCESSFUL!"); Console.WriteLine("##############################################"); Console.WriteLine("Unexpected error:"); Console.WriteLine("##############################################"); PrintException(ex); } finally { Console.WriteLine("Press any key to continue..."); Console.ReadKey(); } } } private static async Task<GroupSummary> GetGroupSummary(long organisationGroupId, string apiBaseUrl, IdServerResourceOwnerClientSettings idServerResourceOwnerClientSettings) { Console.WriteLine("Retrieving Organisation details"); var groupsClient = new GroupsClient(apiBaseUrl, idServerResourceOwnerClientSettings); var group = await groupsClient.GetSubGroupsAsync(organisationGroupId); return group; } private static void PrintException(Exception ex) { Console.WriteLine(ex.Message); if (ex.InnerException != null) PrintException(ex.InnerException); } } }
Useful links
If you prefer to download this, and other samples of the MiX.Integrate REST APIs from Github you can follow the below link.
https://github.com/MiXTelematics/MiX.Integrate.Samples